https://picperf.io/https://laravelnews.s3.amazonaws.com/featured-images/laravel-11-uri-featured.jpg
Laravel 11.35 introduced the Uri
class powered by the PHP League’s URI library. Uri makes it easy to manipulate and work with URIs in your Laravel application and includes some conveniences around named routes.
Basic Manipulation
At the heart of the Uri class is creating and manipulating URI strings, including the query, fragment, and path:
use Illuminate\Support\Uri;
$uri = Uri::of('https://laravel-news.com')
->withPath('links')
->withQuery(['page' => 2])
->withFragment('new');
(string) $url; // https://laravel-news.com/links?page=2#new
$uri->path(); // links
$uri->scheme(); // https
$uri->port(); // null
$uri->host(); // laravel-news.com
Also, note the difference between getting the URI value and decoding the URI:
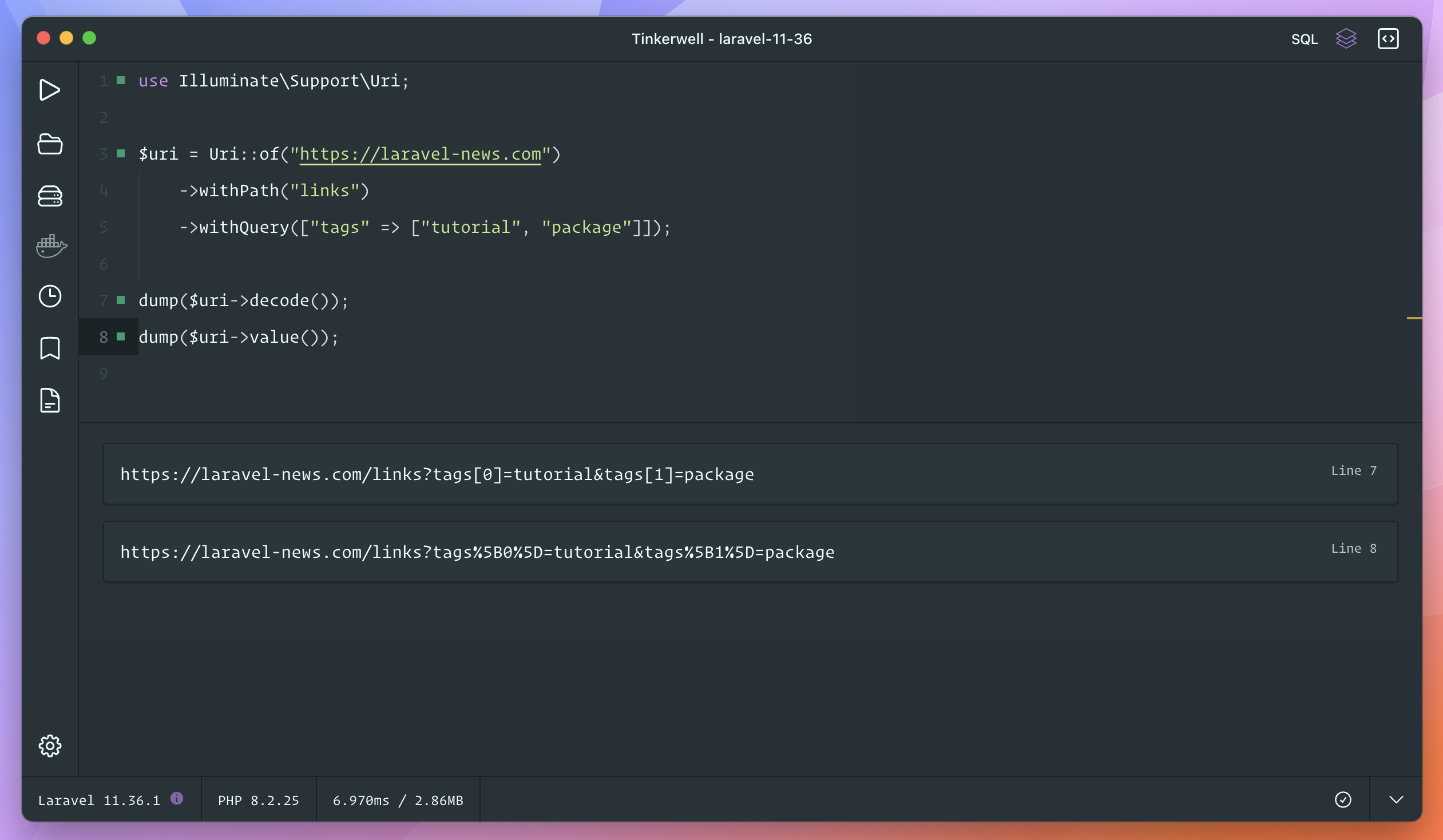
Query Assertion and Manipulation
Asserting and manipulating the URI query params has never been easier in Laravel, using the accompanying UriQueryString
under the hood. The UriQueryString
class uses the support trait InteractsWithData
, which gives you a bunch of useful methods for asserting a query string:
use Illuminate\Support\Uri;
$uri = Uri::of("https://laravel-news.com")
->withPath("links")
->withQuery(["page" => 2, 'name' => ''])
->withFragment("new");
$uri->query()->all(); // ["page" => "2"]
$uri->query()->hasAny("page", "limit"); // true
$uri->query()->has("name"); // true
$uri->query()->has('limit'); // false
$uri->query()->missing('limit'); // true
$uri->query()->filled('page'); // true
$uri->query()->filled("name"); // false
$uri->query()->isNotFilled("name"); // true
$uri->query()->isNotFilled("page"); // false
$uri->query()->string("page", "1"); // Stringable{ value: 2 }
$uri->query()->integer("limit", 10); // 10
Learn about all the useful methods that InteractsWithData provides to the UriQueryString
instances to assert and manipulate query data.
Get an Uri Instance from Named Routes, Paths, and the Current Request
The Uri
class can also create a URI from a named route in your application, a relative URL, or even from the current Request
instance:
// Using a named route
(string) Uri::route("dashboard"); // http://laravel.test/dashboard
// Using a root-relative URL
(string) Uri::to("/dashboard"); // http://laravel.test/dashboard
// From the current request
function (Request $request) {
(string) $request->uri(); // http://laravel.test/dashboard
}
As of Laravel 11.36, the Uri
class is aliased by default in Laravel applications, meaning you can use it without importing the Illuminate\Support\Uri
namespace.
Learn More
We hope you enjoy using Uri
in your Laravel applications! The Uri
class was released in Laravel 11.35 in #53731. Also, read up on InteractsWithData, which provides a ton of useful methods for working with the Uri
class, the Fluent class, and Laravel’s HTTP Request class (via InteractsWithInput
).
The post Working With URIs in Laravel appeared first on Laravel News.
Join the Laravel Newsletter to get all the latest
Laravel articles like this directly in your inbox.
Laravel News