https://s.w.org/images/core/emoji/14.0.0/72×72/1f4a1.png
Are you looking to harness the power of language models for your applications? LangChain, a Python framework, offers a fantastic solution to build applications powered by large language models (LLMs). In this tutorial, we’ll guide you through the essentials of using LangChain and give you a firm foundation for developing your projects.
You’ll begin your journey by learning how to install and set up LangChain, ensuring you have the most up-to-date version. Next, we’ll dive deeper into the core concepts of creating chains, adding components, and running them. By understanding the fundamentals of sequential and custom chains, you’ll be well-equipped to tackle more advanced use cases surrounding LLMs.
By the end, you’ll have a solid understanding of LangChain and be ready to confidently implement it in your Python projects. So, let’s get started to unlock the potential of language models together!
Getting Started with Langchain

Installation
To begin your journey with Langchain, make sure you have a Python version of ≥ 3.8.1 and <4.0. To install the Langchain Python package, simply run the following command:
pip install langchain
This will install the necessary dependencies for you to experiment with large language models using the Langchain framework.
You should also set up Python’s OpenAI integration if you want to use the GPT language models:
Recommended: Python OpenAI API Cheat Sheet
Setup and Configuration
- API Key: Before diving into Langchain tutorials, you’ll need to secure your OpenAI API key. This key allows you to access language models like ChatGPT in various environments. Store your
openai_api_key
safely, as it’s essential for using tools and modules within Langchain. - Imports: Import the necessary Langchain modules to start working with large language models in your Python code. Some commonly used imports include:
from langchain import ConversationChain, Tool
- Creating tools: Tools are functions designed to interact with LLMs (Large Language Models) and handle tasks like Google Search or Database Lookup. You can create custom tools as well:
def custom_tool(input_text: str): # Your code goes here
- Model Configuration: Specify the model name for your application, such as
chatgpt
, and configure settings likememory
, which control the context size and model performance.
model_name = "chatgpt" memory = 4096
- ConversationChain: Harrison Chase devised the
ConversationChain
module to leverage the power of LLMs within a conversation context. You can create instances of this module and use it in various applications like chatbots or generative question-answering.
chain = ConversationChain(model_name=model_name, memory=memory)
With these steps completed, you’re now equipped to explore the world of Langchain and build applications powered by large language models.
Working with LangChain Components

In this section, we will explore various components of LangChain to help you better understand how to effectively use them while working with Large Language Models (LLMs).
Prompt Templates
When working with LangChain, prompt engineering is an essential aspect to get the desired outputs from your LLMs. One important method is to use Prompt Templates. A Prompt Template is a skeleton that structures your input for the language model, making it easier to produce the desired output.
You can define prompt templates by creating a PromptTemplate
object and specifying its input_variables
. Here’s a simple example:
from langchain import PromptTemplate, utils template = PromptTemplate("Translate the following text from English to French: ", input_variables=['text']) translated_text = template.fill({"text": "Hello, how are you?"})
This will generate a translation prompt for the English text input.
Conversation Chains
LangChain also simplifies the creation and management of conversation chains with LLMs. Conversation Chains are designed to make interactions with your LLM more coherent and engaging. You can create a conversation chain using the LLMChain
class:
from langchain import LLMChain, ConversationInput chain = LLMChain(prior_messages=[]) chain.add(ConversationInput(role="user", content="What is the meaning of life?"))
Adding a new input to the chain is as simple as using the add
method, and you can provide either user input or system responses to build an interactive conversation experience.
Language Model Session
While working with LangChain, you’ll often need to manage interactions with the LLM. LangChain makes it easy to maintain a consistent context throughout the session. You can create a new session using the LLMSession
class:
from langchain import LLMSession, utils llm_session = LLMSession(tokenizer=utils.get_language_tokenizer("en"))
When using a session, remember to update the context object with the chosen LLM responses. You can extract tokens, texts, or semantic completions depending on your application needs.
Building Functional Chatbots

Building chatbots with LangChain is a great way to leverage powerful tools like OpenAI’s large language models (LLMs) in your Python applications. In this section, you’ll learn about customizing chat models and improving chatbot responsiveness.
Chat Model Customization
To stand out, your chatbot needs its unique touch. You can customize chat models by importing the langchain
package and configuring the ConversationChain
module, which provides a convenient interface to manage conversational flow.
Here are some customization options:
- Adjust temperature: By tweaking the model’s temperature value, you can control the randomness of your chatbot’s responses. A lower value makes your chatbot more focused and deterministic, while a higher value increases creativity.
- Limit response length: Manage the length of replies by setting a
max_tokens
limit. This ensures the chatbot provides concise responses, but be cautious not to set it too low, or the output may be cut off and appear nonsensical.
For example, here’s a Python snippet for customizing your chatbot:
import langchain conv_chain = langchain.ConversationChain( temperature=0.7, max_tokens=100 ) # ... continue with chatbot implementation
Improving Chatbot Responsiveness
LLMs can sometimes be verbose or slow, so some techniques can improve your chatbot’s responsiveness:
- Use context: Keep track of previous turns and adjust the context for better responses. This can be achieved using the
ConversationChain
module, which maintains a history of interactions and feeds them to the model as needed. - Optimize prompts: Craft more specific prompts for better guidance, focusing the model to generate appropriate replies.
- Cache results: To speed up interactions, consider caching previous query results, which saves time by avoiding repetitive calculations.
In short, integrating LangChain’s ConversationChain
module into your Python projects helps you to build powerful and customizable chatbots while utilizing OpenAI’s agents and large language models. With a little customization, your chatbot can provide unique and engaging conversations for users.
Enhancing LLM Capabilities
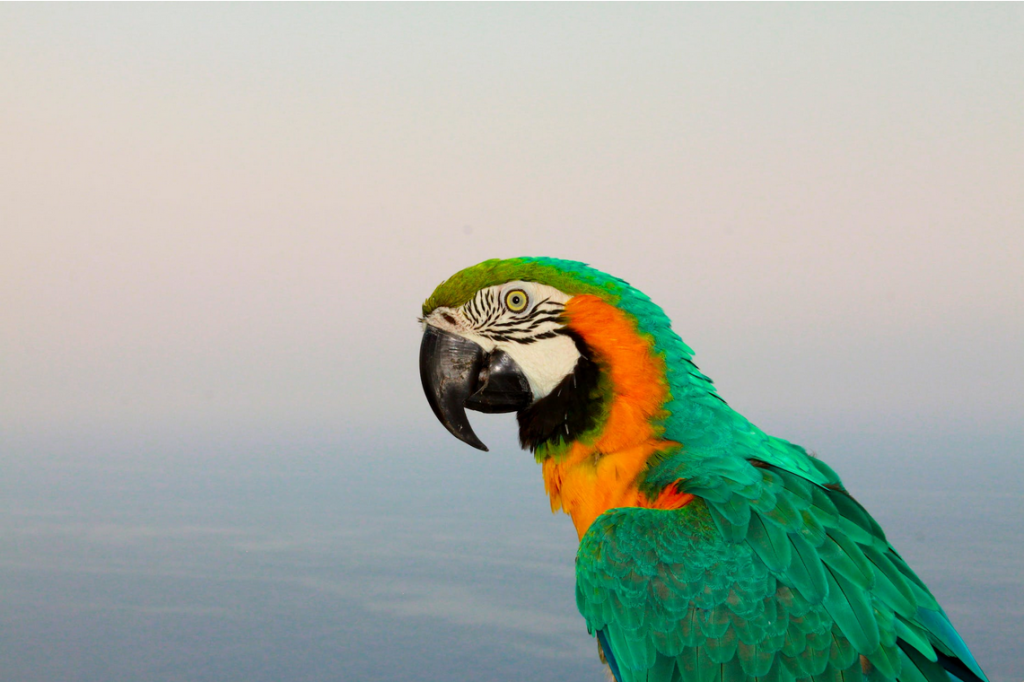
In this section, we will explore how to enhance the capabilities of Language Learning Models (LLMs) using LangChain. We will discuss memory management and expanding language models.
Memory Management
One of the essential aspects of working with LLMs is managing their memory usage. As you work with large models, such as those from Hugging Face or the OpenAI SDK, you might encounter memory limitations. To help with this, LangChain provides several tools.
First, consider using smaller language models when possible. For example, instead of using OpenAI’s text-davinci-003
model, choose a more compact version that still provides good performance.
If memory constraints persist, look into leveraging FAISS (Facebook AI Similarity Search) to perform indexing and search on large text datasets. This allows you to retrieve relevant information from the dataset without loading the entire model.
Expanding Language Models
As you develop your LLM-powered applications, you might want to expand their capabilities by incorporating additional language models. LangChain allows you to easily do this by integrating with platforms like HuggingFace Hub and the OpenAI SDK.
To add a new language model, follow these steps:
- Choose a suitable model from the HuggingFace Hub or the OpenAI SDK based on your application requirements.
- Import the chosen model into your LangChain project.
- Adjust your prompt function to work with the new model.
By exploring different LLMs, you can enhance the capabilities of your application while keeping memory usage under control.
Remember, it’s essential to stay up-to-date with the latest developments in the world of LLMs, so keep learning and experimenting with new models and techniques. And as always, stay curious and have fun exploring the vast possibilities of LLMs with LangChain!
Real-World NLP Use Cases
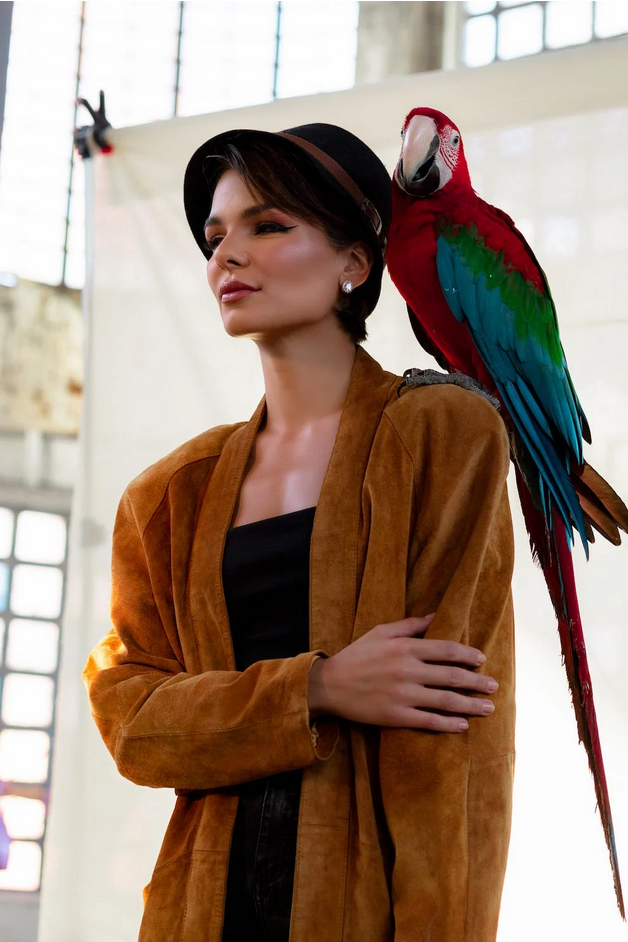
Question Answering Applications
You can leverage large language models (LLMs) and LangChain, a python
library, to build question-answering applications for various domains such as finance, healthcare, or education. These applications can process natural language queries and generate responses by analyzing unstructured text data from multiple sources like Google Search or document loaders.
Structuring input and output data into embeddings helps improve the application’s performance. Use the LangChain library to seamlessly integrate Python REPL and other programming language modules with your custom-built application.
Summarization and Content Generation
Another exciting use case for LLMs and LangChain in real-world NLP is content generation and summarization. Whether it’s creating personalized news feeds or automatically generating high-quality articles, you can achieve this by utilizing LangChain’s capabilities.
To make your application generate comprehensive yet concise summaries, consider using reinforcement learning techniques, data sources, and pre-built text splitters. Your application can even produce real-time content tailored to user preferences, making it a game-changer in the content creation industry.
Natural Language Understanding
Building a deeper understanding of natural language in your application is crucial for numerous NLP tasks. With the help of LLMs and LangChain, you can enhance natural language understanding (NLU) to improve sentiment analysis, customer support automation, or even personal assistant applications.
Efficiently combine information from unstructured text sources to identify patterns and provide better solutions in a user-friendly environment. By incorporating the capabilities of LangChain’s python
library, you can significantly enhance your application’s performance in various natural language processing tasks.
Advanced Techniques and Applications

In this section, we will explore advanced techniques and applications of LangChain that will help you harness the full power of language models for your projects. We will discuss Environmental Context Injection and Math and Reasoning Puzzles in more detail.
Environmental Context Injection
With Environmental Context Injection, you can provide context to your language models, such as with bloom
or llama
, to improve their understanding and responses. To leverage this feature, use LangChain’s prompt management and prompt templates for easier and more efficient context management.
For example, if you want to look up information from a database, you can use a combination of database lookup and prompt templates to automatically generate the correct prompt for your language model. This can be done with a zero-shot-react-description or by using a vector store such as vectorstore
.
Math and Reasoning Puzzles
LangChain can also help you create and solve math and reasoning puzzles using built-in memory capabilities. To create a math puzzle, follow these steps:
- Design a prompt template to describe the puzzle that your language model should solve.
- Use the prompt management system to add any necessary context or information needed to solve the puzzle.
- Leverage the powerful memory capabilities of your language model to come up with a solution.
Here’s an example of a simple math prompt template:
Solve the following mathematical problem:
1. Problem: 2 x (3 + 4)
2. Target Answer: 14
Can you show the step-by-step solution to this problem?
In this example, the language model will provide you with the step-by-step solution to the problem. By utilizing LangChain for math and reasoning puzzles, you can create engaging applications that harness the cognitive abilities of advanced language models.
With these advanced techniques and the right prompt management approach, you can unlock a wide range of possibilities for integrating LangChain into your projects, from answering questions based on database lookups to devising creative problem-solving scenarios. Enjoy exploring these powerful features and enhancing your language-based applications.
Resources and References

Langchain Documentation
For a comprehensive guide on LangChain, you can refer to its official documentation, which provides valuable information on installing, setting up your environment, and working with this useful Python framework. The documentation covers various components, like different supported models for machine learning, memory management, and more.
APIs and Third-Party Integration
LangChain allows you to integrate with various APIs, such as OpenAI’s GPT-3 and other LLM providers.
Recommended: 11 Best ChatGPT Alternatives
For instance, you can connect it with Flan-T5 for tasks like summarization. To interact with GPT-3 and GPT-4, you can connect it to the ChatOpenAI API. This integration helps you to build powerful language processing applications.
Additionally, LangChain works with external data sources like SerpApi for retrieving search engine result pages, and supports data management with various databases. Persistent state storage is possible through the ConversationBufferMemory component.
Indexes and Vector Databases
LangChain can also integrate with vector databases, like Pinecone’s vector database, to provide efficient and scalable storage for high-dimensional vectors. This is useful when working with LLMs as it enables advanced use cases such as similarity search or clustering.
Setting up your environment to work with LangChain, including the required environment variables, is crucial for smooth operation. Make sure to follow the Langchain installation guide to set up the required environment variables and input parameters.
Feel free to also check out our cheat sheets by subscribing to our email newsletter (100% free):
OpenAI Glossary Cheat Sheet (100% Free PDF Download) 
Finally, check out our free cheat sheet on OpenAI terminology, many Finxters have told me they love it!
Recommended: OpenAI Terminology Cheat Sheet (Free Download PDF)
Be on the Right Side of Change