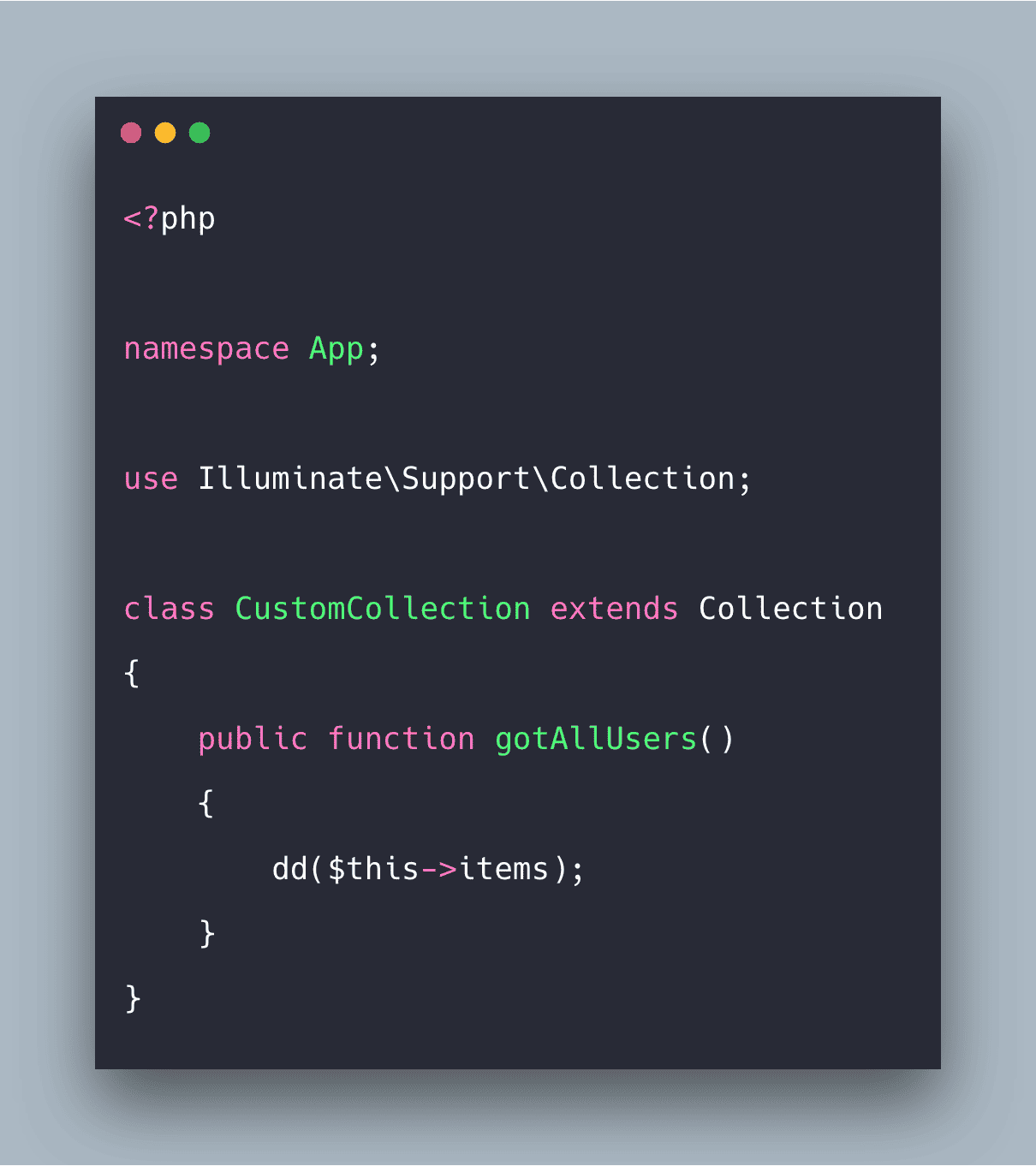
Laravel Eloquent Collection Tutorial With Example is today’s topic. The Eloquent collection object extends the Laravel base collection, so it naturally inherits the dozens of methods used to work with an underlying array of Eloquent models fluently. All multi-result sets returned by Eloquent are instances of an Illuminate\Database\Eloquent\Collection object, including results retrieved via the get method or accessed via a relationship.
Laravel Eloquent Collection Tutorial
We will start this tutorial by installing fresh Laravel. Right now, Laravel 5.8 is the latest version of Laravel. If you are new to Laravel 5.8, then check out my Laravel 5.8 CRUD tutorial for starters. Install Laravel using the following command.
composer create-project --prefer-dist laravel/laravel blog
Okay, now set up the database inside the .env file.
DB_CONNECTION=mysql DB_HOST=127.0.0.1 DB_PORT=3306 DB_DATABASE=blog DB_USERNAME=root DB_PASSWORD=root
Next step is to migrate the tables inside the database using the following command.
php artisan migrate
Seed Database
Laravel includes the simple method of seeding your database with test data using seed classes. All the seed classes are stored in the database/seeds directory. Seed classes may have any name you want but probably it should follow some sensible convention, such as UsersTableSeeder, etc. By default, the DatabaseSeeder class is defined for you. From this class, you can use the call() method to run other seed classes, allowing you to control the seeding order.
Create a UsersTableSeeder.php file using the following command.
php artisan make:seeder UsersTableSeeder
We will use Model factories to generate fake data.
Now, write the following code inside the UsersTableSeeder.php file.
<?php // UsersTableSeeder.php use Illuminate\Database\Seeder; use Illuminate\Support\Str; class UsersTableSeeder extends Seeder { /** * Run the database seeds. * * @return void */ public function run() { $faker = Faker\Factory::create(); for($i=0;$i<50;$i++) { \App\User::create([ 'name' => $faker->name, 'email' => $faker->unique()->safeEmail, 'email_verified_at' => now(), 'password' => '$2y$10$TKh8H1.PfQx37YgCzwiKb.KjNyWgaHb9cbcoQgdIVFlYg7B77UdFm', // secret 'remember_token' => Str::random(10), ]); } } }
So, it will generate 50 random users records in the database.
Now, we need to modify the DatabaseSeeder.php file.
<?php // DatabaseSeeder.php use Illuminate\Database\Seeder; class DatabaseSeeder extends Seeder { /** * Seed the application's database. * * @return void */ public function run() { $this->call(UsersTableSeeder::class); } }
Before you seed the file, we need to regenerate the Composer’s autoloader using the dump-autoload command.
composer dump-autoload
Okay, now go to the terminal and run the seed file using the following command.
php artisan db:seed
It will generate fake data in the users table.
So now, we have test data to work with, and we can query the database using Laravel Eloquent Collection.
Query Data using Laravel Eloquent Collection
Now, write the following code inside the routes >> web.php file.
<?php // web.php Route::get('/', function () { $users = \App\User::all(); foreach ($users as $user) { echo '<pre>'; echo $user->name; echo '</pre>'; } });
In the above code, we are displaying only the name of the users in preformatted HTML view.
If you go to the root route, then you will see something like below image. Of course, the data will be different because the faker library randomly generates it. It will be 50 names.
All collections also serve as the iterators, allowing us to loop over them as if they were simple PHP arrays.
The collections are much more potent than arrays and expose the variety of map / reduce operations that may be chained using the intuitive interface.
Laravel chunk() Collection Method
The chunk() method breaks a collection into multiple, smaller collections of the given size.
Write the following code inside the web.php file.
<?php // web.php Route::get('/', function () { $users = \App\User::all(); $chunks = $users->chunk(2); $data = $chunks->toArray(); echo '<pre>'; print_r($data); echo '</pre>'; });
The output of the above code is following.
The chunk() method is especially useful in views when working with the grid system such as Bootstrap.
Laravel Custom Collections
If you need to use the custom Collection object with your extension methods, you may override the newCollection method on your model. See the following example of the User.php model.
<?php // User.php namespace App; use Illuminate\Notifications\Notifiable; use Illuminate\Contracts\Auth\MustVerifyEmail; use Illuminate\Foundation\Auth\User as Authenticatable; use App\CustomCollection; class User extends Authenticatable { use Notifiable; /** * The attributes that are mass assignable. * * @var array */ protected $fillable = [ 'name', 'email', 'password', ]; /** * The attributes that should be hidden for arrays. * * @var array */ protected $hidden = [ 'password', 'remember_token', ]; /** * The attributes that should be cast to native types. * * @var array */ protected $casts = [ 'email_verified_at' => 'datetime', ]; /** * Create a new Eloquent Collection instance. * * @param array $models * @return \Illuminate\Database\Eloquent\Collection */ public function newCollection(array $models = []) { return new CustomCollection($models); } }
Once you have defined the newCollection method, you will receive an instance of your custom collection anytime Eloquent returns the collection instance of that model. If you would like to use the custom collection for every model in your application, you should override a newCollection method on the base model class that is extended by all of your models. In the above example, we have override inside the User.php model class.
Okay, now create a CustomCollection.php file inside the app folder.
<?php // CustomCollection.php namespace App; use Illuminate\Support\Collection; class CustomCollection extends Collection { public function gotAllUsers() { dd($this->items); } }
Here, $this->items have all the users records. We can access these records inside the CustomCollection class.
Finally, write the following code inside the web.php file.
<?php // web.php Route::get('/', function () { $users = \App\User::get(); $users->gotAllUsers(); });
Refresh the root route, and the output is following. It is up to 50 records.
Finally, Laravel Eloquent Collection Tutorial With Example is over.
The post Laravel Eloquent Collection Tutorial With Example | Laravel 5.8 Guide appeared first on AppDividend.
via Planet MySQL
Laravel Eloquent Collection Tutorial With Example | Laravel 5.8 Guide