https://blog.finxter.com/wp-content/uploads/2021/08/image-33.png
When it comes to developing web applications in Python there are lots of frameworks. Some examples are Pyramid, Web2Py, Bottle or CherryPy, among others. However, the two most popular ones are Flask and Django.
We can confirm this fact by having a look at the most starred Python libraries in GitHub:
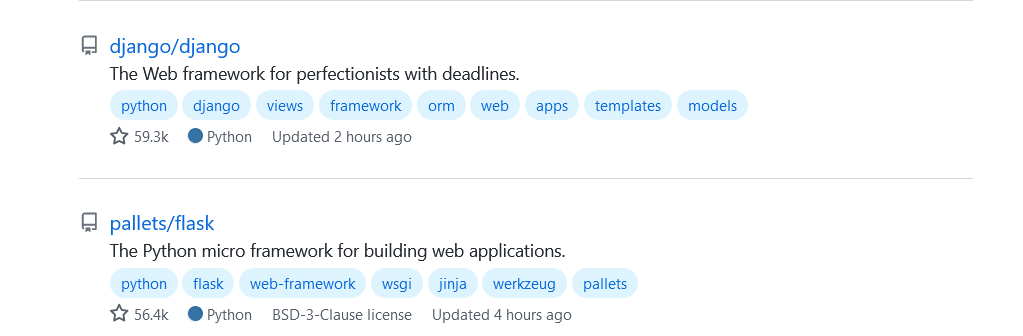
As we will see in this post, both of these frameworks follow very different design principles. Thus, we can not conclude that one is superior to the other. To choose the one that is best for you comes down to which type of application you want to build. In this article we will deep in the advantages and disadvantages of both of these frameworks. Therefore, you can make an informed decision on which one better fits your needs.
What is Flask?
Flask is a microframework conceived to develop web applications in Python. It started in 2010 as an April Fools Day Joke. The key concept in Flask is the word “micro” that refers to the fact that its core is simple but extensible. Because of that it can be learned fast and has a good learning curve. For instance, the Flask hello world app can be written in just five lines of code.
from flask import Flask app = Flask(__name__) @app.route("/") def hello_world(): return "<p>Hello, World!</p>"
And to initialize the server you must type the following command in the terminal, which runs the app by default on the URL http://127.0.0.1:5000.
$ FLASK_APP=hello.py flask run
Flask Advantages and Drawbacks
Flask has been designed to start web development quickly and easily. This makes it ideal for building prototype applications. The word that best defines it is “minimalism”, as all that Flask includes are four main components: a template engine (named Jinja2), URL routing, error handling and a debugger. That means that a Flask application is lightweight. In turn, as it does not have to run a lot of code it is also a little bit faster than Django.
But Flask’s minimalism does not mean that you can’t build great web applications with it. Instead Flask is flexible, like a Lego construction set. All the functionalities expected from a web application like an Object-Relational mapping (ORM), security, authentication, data validation, etc. are delegated to third-party libraries that you must choose on. Moreover, there are extensions like Flask-Security that bundle together security libraries that people typically use with Flask.
However, Flask’s flexibility comes with many drawbacks. First, two Flask applications can follow very different patterns, which means that it can be hard for a developer to switch from one to another. Second, as Flask’s extensions are developed by different teams, a Flask app is more prone to security risks and requires more time to keep it updated. Last, because of the use of different libraries the documentation that you can find is very spread over the internet.
What is Django?
Django is a “full stack” web framework that can tackle scalable and high quality web applications. It was publicly released in 2005, but it started earlier as a Python CMS at the Lawrence Journal-World newspaper. In contrast with Flask, Django forces you to do things their own way. Thus, it has a steeper learning curve and can be more intimidating to beginners.
A Django application involves at least the use of three files, and four when a data model is involved. To create a hello world application with Django is as follows.
First, we create a Django project in a folder called config
.
$ django-admin startproject config .
We then create an app named pages by typing the following command. This creates a pages folder, located at the same level of the config folder, containing different files.
$ python manage.py startapp pages
In the next step we update the file pages/views.py
to make it look as follows:
from django.http import HttpResponse def helloPageView(request): return HttpResponse("Hello, World!")
Then we update the file pages/urls.py
with the following code:
from django.urls import path from .views import helloPageView urlpatterns = [ path('', helloPageView, name='home') ]
The last file to update is the config/urls.py
file:
from django.contrib import admin from django.urls import path, include urlpatterns = [ path('admin/', admin.site.urls), path('', include('pages.urls')) ]
Finally, we can run our Django hello world application with the following command, which starts the server on the URL http://127.0.0.1:8000.
$ python manage.py runserver
As we have seen in this example, the hello world Django application involves much more steps than the same application with Flask.
Django Advantages and Drawbacks
Django follows the principle of “Don’t Repeat Yourself” and includes all the components that are needed to build a web application. It has out of the box templating, forms, routing, authentication, database administration among other features. It requires less decisions to be made by you. Because of that, an experienced Python developer who wants to dig into web development can do it in a relatively short amount of time. This also means that Django projects follow a pattern making them very similar one to another.
When you use Django you are forced to use its ORM, which assumes that you are going to use a relational database. It officially supports databases like MariaDB, MySQL or SQLite among others. With Django’s ORM you can do almost every operation that an ordinary web app needs. In addition to that, it allows you to write your own SQL queries. The negative side of it is that you can forget about using a NoSQL database like MongoDB with Django.
In terms of security, Django is very reliable as it incorporates features like protection against SQL injection and cross site scripting (XSS) attacks, the possibility to enable HTTPS protection, or JSON web tokens (through external libraries). So it is very suitable for applications that require their users to authenticate or that involve monetary transactions.
Another great aspect of Django is its community. As a popular web framework, Django has a very big community with lots of documentation available online. For instance it has more than 200k tagged questions on Stack Overflow.
But the Django way of doing things, handling everything for you, can also be a drawback as it produces monolithic servers that act as a single unit. This means that it is not possible to develop a microservice architecture with Django.
Differences Side by Side
As a summary these are the main differences between Flask and Django.
Flask | Django | |
Type of framework | Microframework with lightweight code | Full stack, provides everything you need |
Learning | Easy to learn | Steeper learning curve |
Project size | For smaller and less complicated projects | For larger projects |
Project layout | Arbitrary | Follows a pattern |
Templates | Relies on Jinja2 engine | Built-in engine |
Databases | Lack of ORM but can work with many different databases through libraries | Own ORM for relational databases like MariaBD, MySQL, SQLite, etc. |
Admin | Can be done with the Flask-Admin extension | Built-in panel for admin tasks |
Security | Depends on the security of external libraries | Built-in security features |
API | Supports API | Does not support API |
Flexibility | Very flexible, allowing developers to add their own libraries | Low, developers must follow Django’s rules |
Performance | Slightly better | Sufficient for any application |
What in Terms of Freelance Projects?
As you might know, Finxter.com promotes remote work by helping people to start their careers as Python freelance developers. Two well established websites where freelancers can apply to projects posted by companies are Freelancer.com and Upwork.
At the time of writing, we can find at Freelancer.com 82 projects when we search for the keyword Django and 14 projects when we search for Flask.
If we look at Upwork the number of projects are higher for both frameworks. In particular, we find 717 Django projects and 336 Flask projects.
We see that Upwork has a substantially higher number of projects to apply in terms of Python web development. We also see a higher number of Django projects on both websites .
However, the above numbers are snapshots and can vary depending on the time we take them. But if we look at time series like worldwide searches of Flask and Django in the last five years, provided by Google trends, we can confirm that there is a higher interest in Django.
When to Use One or the Other?
As we have seen in this post Flask and Django are two sides of the same coin. Now that we know all that they offer, this is my recommendation on which cases are best to use one or the other.
Better to use Flask if:
- You are new to Python.
- You just want to build a simple prototype application.
- You want to know how your application works and operates internally.
- Your application is based on NoSQL.
- You plan to build a microservice architecture.
- You want to build simple web apps like REST APIs, IoT apps or small websites with static content.
But you better use Django if:
- You are already experienced in Python.
- Your application will use a SQL database.
- Your application will have users that need to be authenticated.
- You want an application with user administration panels.
- Your application will serve dynamic content.
The post Flask vs Django: Comparing the Two Most Popular Python Web Frameworks first appeared on Finxter.Finxter