https://s.w.org/images/core/emoji/14.0.0/72×72/1f447.png
Since I frequently handle textual data with Python , I’ve encountered the challenge of converting lists of strings into different data types time and again. This article, originally penned for my own reference, decisively tackles this issue and might just prove useful for you too!
Let’s get started!
Python Convert List of Strings to Ints
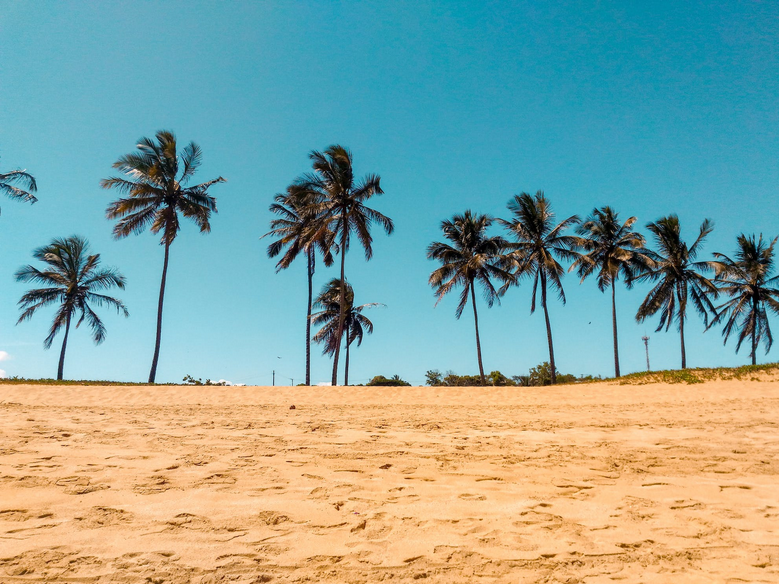
This section is for you if you have a list of strings representing numbers and want to convert them to integers.
The first approach is using a for
loop to iterate through the list and convert each string to an integer using the int()
function.
Here’s a code snippet to help you understand:
string_list = ['1', '2', '3'] int_list = [] for item in string_list: int_list.append(int(item)) print(int_list) # Output: [1, 2, 3]
Another popular method is using list comprehension. It’s a more concise way of achieving the same result as the for
loop method.
Here’s an example:
string_list = ['1', '2', '3'] int_list = [int(item) for item in string_list] print(int_list) # Output: [1, 2, 3]
You can also use the built-in map()
function, which applies a specified function (in this case, int()
) to each item in the input list. Just make sure to convert the result back to a list using list()
.
Take a look at this example:
string_list = ['1', '2', '3'] int_list = list(map(int, string_list)) print(int_list) # Output: [1, 2, 3]
For a full guide on the matter, check out our blog tutorial:
Recommended: How to Convert a String List to an Integer List in Python
Python Convert List Of Strings To Floats

If you want to convert a list of strings to floats in Python, you’ve come to the right place. Next, let’s explore a few different ways you can achieve this.
First, one simple and Pythonic way to convert a list of strings to a list of floats is by using list comprehension.
Here’s how you can do it:
strings = ["1.2", "2.3", "3.4"] floats = [float(x) for x in strings]
In this example, the list comprehension iterates over each element in the strings
list, converting each element to a float using the built-in float()
function.
Another approach is to use the map()
function along with float()
to achieve the same result:
strings = ["1.2", "2.3", "3.4"] floats = list(map(float, strings))
The map()
function applies the float()
function to each element in the strings
list, and then we convert the result back to a list using the list()
function.
If your strings contain decimal separators other than the dot (.
), like a comma (,
), you need to replace them first before converting to floats:
strings = ["1,2", "2,3", "3,4"] floats = [float(x.replace(',', '.')) for x in strings]
This will ensure that the values are correctly converted to float numbers.
Recommended: How to Convert a String List to a Float List in Python
Python Convert List Of Strings To String
You might need to convert a list of strings into a single string in Python. It’s quite simple! You can use the
join()
method to combine the elements of your list.
Here’s a quick example:
string_list = ['hello', 'world'] result = ''.join(string_list) # Output: 'helloworld'
You might want to separate the elements with a specific character or pattern, like spaces or commas. Just modify the string used in the join()
method:
result_with_spaces = ' '.join(string_list) # Output: 'hello world' result_with_commas = ', '.join(string_list) # Output: 'hello, world'
If your list contains non-string elements such as integers or floats, you’ll need to convert them to strings first using a list comprehension or a map()
function:
integer_list = [1, 2, 3] # Using list comprehension str_list = [str(x) for x in integer_list] result = ','.join(str_list) # Output: '1,2,3' # Using map function str_list = map(str, integer_list) result = ','.join(str_list) # Output: '1,2,3'
Play around with different separators and methods to find the best suits your needs.
Python Convert List Of Strings To One String
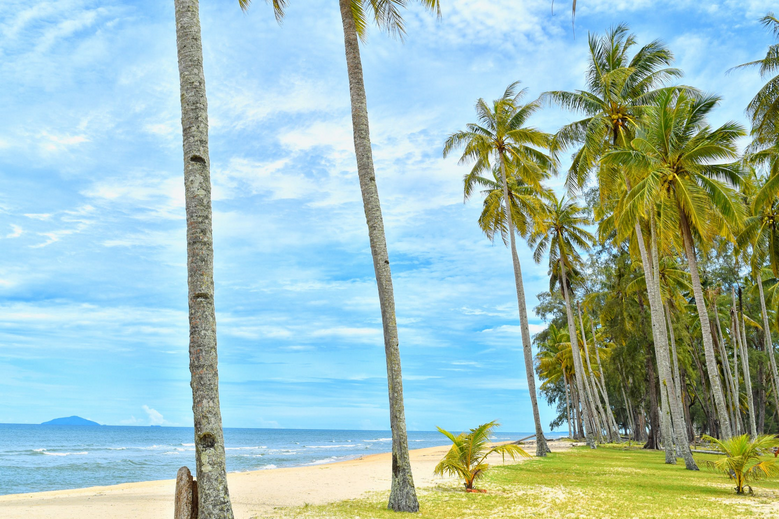
Are you looking for a simple way to convert a list of strings to a single string in Python?
The easiest method to combine a list of strings into one string uses the join()
method. Just pass the list of strings as an argument to join()
, and it’ll do the magic for you.
Here’s an example:
list_of_strings = ["John", "Charles", "Smith"] combined_string = " ".join(list_of_strings) print(combined_string)
Output:
John Charles Smith
You can also change the separator by modifying the string before the join()
call. Now let’s say your list has a mix of data types, like integers and strings. No problem! Use the map()
function along with join()
to handle this situation:
list_of_strings = ["John", 42, "Smith"] combined_string = " ".join(map(str, list_of_strings)) print(combined_string)
Output:
John 42 Smith
In this case, the map()
function converts every element in the list to a string before joining them.
Another solution is using the str.format()
method to merge the list elements. This is especially handy when you want to follow a specific template.
For example:
list_of_strings = ["John", "Charles", "Smith"] result = " {} {} {}".format(*list_of_strings) print(result)
Output:
John Charles Smith
And that’s it! Now you know multiple ways to convert a list of strings into one string in Python.
Python Convert List of Strings to Comma Separated String
So you’d like to convert a list of strings to a comma-separated string using Python.
Here’s a simple solution that uses the join()
function:
string_list = ['apple', 'banana', 'cherry'] comma_separated_string = ','.join(string_list) print(comma_separated_string)
This code would output:
apple,banana,cherry
Using the join()
function is a fantastic and efficient way to concatenate strings in a list, adding your desired delimiter (in this case, a comma) between every element .
In case your list doesn’t only contain strings, don’t sweat! You can still convert it to a comma-separated string, even if it includes integers or other types. Just use list comprehension along with the str()
function:
mixed_list = ['apple', 42, 'cherry'] comma_separated_string = ','.join(str(item) for item in mixed_list) print(comma_separated_string)
And your output would look like:
apple,42,cherry
Now you have a versatile method to handle lists containing different types of elements

Remember, if your list includes strings containing commas, you might want to choose a different delimiter or use quotes to better differentiate between items.
For example:
list_with_commas = ['apple,green', 'banana,yellow', 'cherry,red'] comma_separated_string = '"{}"'.format('", "'.join(list_with_commas)) print(comma_separated_string)
Here’s the output you’d get:
"apple,green", "banana,yellow", "cherry,red"
With these tips and examples, you should be able to easily convert a list of strings (or mixed data types) to comma-separated strings in Python .
Python Convert List Of Strings To Lowercase
Let’s dive into converting a list of strings to lowercase in Python. In this section, you’ll learn three handy methods to achieve this. Don’t worry, they’re easy!
Solution: List Comprehension
Firstly, you can use list comprehension to create a list with all lowercase strings. This is a concise and efficient way to achieve your goal.
Here’s an example:
original_list = ["Hello", "WORLD", "PyThon"] lowercase_list = [item.lower() for item in original_list] print(lowercase_list) # Output: ['hello', 'world', 'python']
With this approach, the lower()
method is applied to each item in the list, creating a new list with lowercase strings.
Solution: map()
Function
Another way to convert a list of strings to lowercase is by using the map()
function. This function applies a given function (in our case, str.lower()
) to each item in a list.
Here’s an example:
original_list = ["Hello", "WORLD", "PyThon"] lowercase_list = list(map(str.lower, original_list)) print(lowercase_list) # Output: ['hello', 'world', 'python']
Remember to wrap the map()
function with the list()
function to get your desired output.
Solution: For Loop
Lastly, you can use a simple for
loop. This approach might be more familiar and readable to some, but it’s typically less efficient than the other methods mentioned.
Here’s an example:
original_list = ["Hello", "WORLD", "PyThon"] lowercase_list = [] for item in original_list: lowercase_list.append(item.lower()) print(lowercase_list) # Output: ['hello', 'world', 'python']
I have written a complete guide on this on the Finxter blog. Check it out!
Recommended: Python Convert String List to Lowercase
Python Convert List of Strings to Datetime

In this section, we’ll guide you through converting a list of strings to datetime
objects in Python. It’s a common task when working with date-related data, and can be quite easy to achieve with the right tools!
So, let’s say you have a list of strings representing dates, and you want to convert this into a list of datetime
objects. First, you’ll need to import the datetime
module to access the essential functions.
from datetime import datetime
Next, you can use the strptime()
function from the datetime
module to convert each string in your list to a datetime object. To do this, simply iterate over the list of strings and apply the strptime
function with the appropriate date format.
For example, if your list contained dates in the "YYYY-MM-DD"
format, your code would look like this:
date_strings_list = ["2023-05-01", "2023-05-02", "2023-05-03"] date_format = "%Y-%m-%d" datetime_list = [datetime.strptime(date_string, date_format) for date_string in date_strings_list]
By using list comprehension, you’ve efficiently transformed your list of strings into a list of datetime
objects!
Keep in mind that you’ll need to adjust the date_format
variable according to the format of the dates in your list of strings. Here are some common date format codes you might need:
%Y
: Year with century, as a decimal number (e.g., 2023)%m
: Month as a zero-padded decimal number (e.g., 05)%d
: Day of the month as a zero-padded decimal number (e.g., 01)%H
: Hour (24-hour clock) as a zero-padded decimal number (e.g., 17)%M
: Minute as a zero-padded decimal number (e.g., 43)%S
: Second as a zero-padded decimal number (e.g., 08)
Python Convert List Of Strings To Bytes
So you want to convert a list of strings to bytes in Python? No worries, I’ve got your back. This brief section will guide you through the process.
First things first, serialize your list of strings as a JSON string, and then convert it to bytes. You can easily do this using Python’s built-in json
module.
Here’s a quick example:
import json your_list = ['hello', 'world'] list_str = json.dumps(your_list) list_bytes = list_str.encode('utf-8')
Now, list_bytes
is the byte representation of your original list.
But hey, what if you want to get back the original list from those bytes? Simple! Just do the reverse:
reconstructed_list = json.loads(list_bytes.decode('utf-8'))
And voilà! You’ve successfully converted a list of strings to bytes and back again in Python.
Remember that this method works well for lists containing strings. If your list includes other data types, you may need to convert them to strings first.
Python Convert List of Strings to Dictionary

Next, you’ll learn how to convert a list of strings to a dictionary. This can come in handy when you want to extract meaningful data from a list of key-value pairs represented as strings.
To get started, let’s say you have a list of strings that look like this:
data_list = ["Name: John", "Age: 30", "City: New York"]
You can convert this list into a dictionary using a simple loop and the split()
method.
Here’s the recipe:
data_dict = {} for item in data_list: key, value = item.split(": ") data_dict[key] = value print(data_dict) # Output: {"Name": "John", "Age": "30", "City": "New York"}
Sweet, you just converted your list to a dictionary! But, what if you want to make it more concise? Python offers an elegant solution with dictionary comprehension.
Check this out:
data_dict = {item.split(": ")[0]: item.split(": ")[1] for item in data_list} print(data_dict) # Output: {"Name": "John", "Age": "30", "City": "New York"}
With just one line of code, you achieved the same result. High five!
When dealing with more complex lists that contain strings in various formats or nested structures, it’s essential to use additional tools like the json.loads()
method or the ast.literal_eval()
function. But for simple cases like the example above, the loop and dictionary comprehension should be more than enough.
Python Convert List Of Strings To Bytes-Like Object
How to convert a list of strings into a bytes-like object in Python? It’s quite simple and can be done easily using the
json
library and the utf-8
encoding.
Firstly, let’s tackle encoding your list of strings as a JSON string . You can use the
json.dumps()
function to achieve this.
Here’s an example:
import json your_list = ['hello', 'world'] json_string = json.dumps(your_list)
Now that you have the JSON string, you can convert it to a bytes-like object using the encode()
method of the string .
Simply specify the encoding you’d like to use, which in this case is 'utf-8'
:
bytes_object = json_string.encode('utf-8')
And that’s it! Your list of strings has been successfully transformed into a bytes-like object. To recap, here’s the complete code snippet:
import json your_list = ['hello', 'world'] json_string = json.dumps(your_list) bytes_object = json_string.encode('utf-8')
If you ever need to decode the bytes-like object back into a list of strings, just use the decode()
method followed by the json.loads()
function like so:
decoded_string = bytes_object.decode('utf-8') original_list = json.loads(decoded_string)
Python Convert List Of Strings To Array

Converting a list of strings to an array in Python is a piece of cake .
One simple approach is using the NumPy library, which offers powerful tools for working with arrays. To start, make sure you have NumPy installed. Afterward, you can create an array using the numpy.array()
function.
Like so:
import numpy as np string_list = ['apple', 'banana', 'cherry'] string_array = np.array(string_list)
Now your list is enjoying its new life as an array!
But sometimes, you may need to convert a list of strings into a specific data structure, like a NumPy character array. For this purpose, numpy.char.array()
comes to the rescue:
char_array = np.char.array(string_list)
Now you have a character array! Easy as pie, right?
If you want to explore more options, check out the built-in split()
method that lets you convert a string into a list, and subsequently into an array. This method is especially handy when you need to split a string based on a separator or a regular expression.
Python Convert List Of Strings To JSON
You’ve probably encountered a situation where you need to convert a list of strings to JSON format in Python. Don’t worry! We’ve got you covered. In this section, we’ll discuss a simple and efficient method to convert a list of strings to JSON using the json
module in Python.
First things first, let’s import the necessary module:
import json
Now that you’ve imported the json
module, you can use the json.dumps()
function to convert your list of strings to a JSON string.
Here’s an example:
string_list = ["apple", "banana", "cherry"] json_string = json.dumps(string_list) print(json_string)
This will output the following JSON string:
["apple", "banana", "cherry"]
Great job! You’ve successfully converted a list of strings to JSON. But what if your list contains strings that are already in JSON format?
In this case, you can use the json.loads()
function:
string_list = ['{"name": "apple", "color": "red"}', '{"name": "banana", "color": "yellow"}'] json_list = [json.loads(string) for string in string_list] print(json_list)
The output will be:
[{"name": "apple", "color": "red"}, {"name": "banana", "color": "yellow"}]
And that’s it! Now you know how to convert a list of strings to JSON in Python, whether it’s a simple list of strings or a list of strings already in JSON format.
Python Convert List Of Strings To Numpy Array
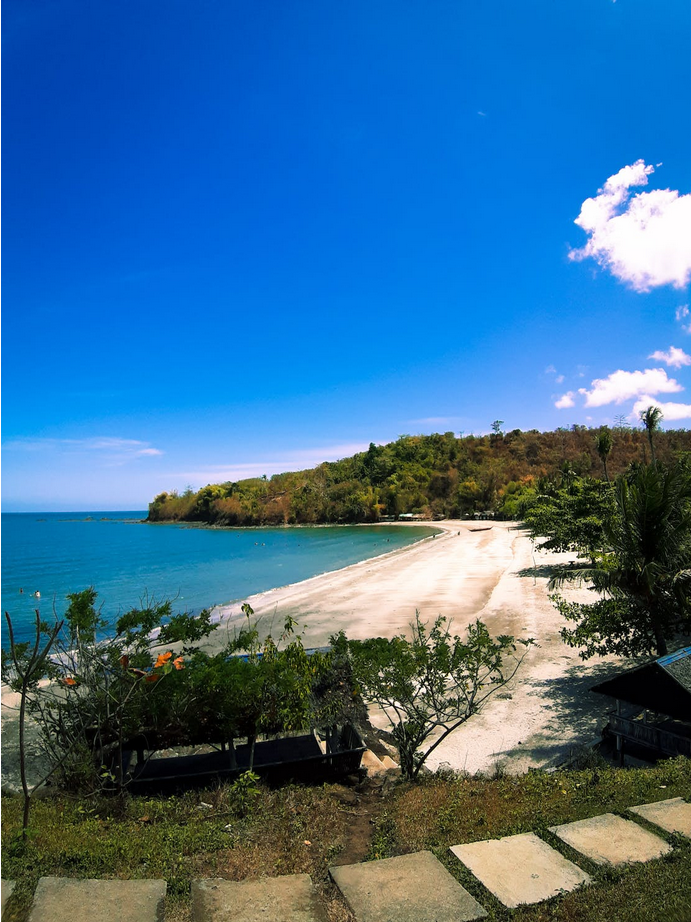
Are you looking to convert a list of strings to a numpy array in Python? Next, we will briefly discuss how to achieve this using NumPy.
First things first, you need to import numpy
. If you don’t have it installed, simply run pip install numpy
in your terminal or command prompt.
Once you’ve done that, you can import numpy
in your Python script as follows:
import numpy as np
Now that numpy
is imported, let’s say you have a list of strings with numbers that you want to convert to a numpy array, like this:
A = ['33.33', '33.33', '33.33', '33.37']
To convert this list of strings into a NumPy array, you can use a simple list comprehension to first convert the strings to floats and then use the numpy array()
function to create the numpy array:
floats = [float(e) for e in A] array_A = np.array(floats)
Congratulations! You’ve successfully converted your list of strings to a numpy array! Now that you have your numpy array, you can perform various operations on it. Some common operations include:
- Finding the mean, min, and max:
mean, min, max = np.mean(array_A), np.min(array_A), np.max(array_A)
- Reshaping the array:
reshaped_array = array_A.reshape(2, 2)
- Performing element-wise operations (e.g., adding):
array_B = np.array([1.0, 2.0, 3.0, 4.0]) result = array_A + array_B
Now you know how to convert a list of strings to a numpy array and perform various operations on it.
Python Convert List of Strings to Numbers
To convert a list of strings to numbers in Python, Python’s map
function can be your best friend. It applies a given function to each item in an iterable. To convert a list of strings into a list of numbers, you can use map
with either the int
or float
function.
Here’s an example:
string_list = ["1", "2", "3", "4", "5"] numbers_int = list(map(int, string_list)) numbers_float = list(map(float, string_list))
Alternatively, using list comprehension is another great approach. Just loop through your list of strings and convert each element accordingly.
Here’s what it looks like:
numbers_int = [int(x) for x in string_list] numbers_float = [float(x) for x in string_list]
Maybe you’re working with a list that contains a mix of strings representing integers and floats. In that case, you can implement a conditional list comprehension like this:
mixed_list = ["1", "2.5", "3", "4.2", "5"] numbers_mixed = [int(x) if "." not in x else float(x) for x in mixed_list]
And that’s it! Now you know how to convert a list of strings to a list of numbers using Python, using different techniques like the map
function and list comprehension.
Python Convert List Of Strings To Array Of Floats
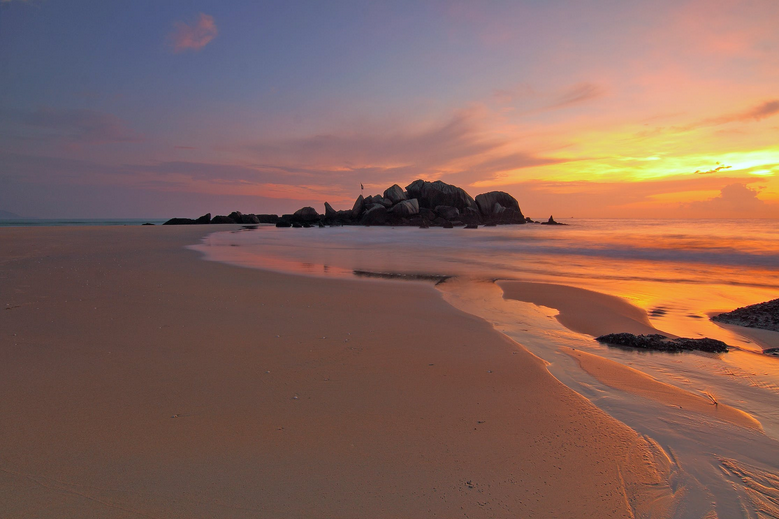
Starting out, you might have a list of strings containing numbers, like
['1.2', '3.4', '5.6']
, and you want to convert these strings to an array of floats in Python.
Here’s how you can achieve this seamlessly:
Using List Comprehension
List comprehension is a concise way to create lists in Python. To convert the list of strings to a list of floats, you can use the following code:
list_of_strings = ['1.2', '3.4', '5.6'] list_of_floats = [float(x) for x in list_of_strings]
This will give you a new list
list_of_floats
containing [1.2, 3.4, 5.6]
.
Using numpy. 
If you have numpy installed or are working with larger arrays, you might want to convert the list of strings to a numpy array of floats.
Here’s how you can do that:
import numpy as np list_of_strings = ['1.2', '3.4', '5.6'] numpy_array = np.array(list_of_strings, dtype=float)
Now you have a numpy array of floats: array([1.2, 3.4, 5.6])
.
Converting Nested Lists
If you’re working with a nested list of strings representing numbers, like:
nested_list_of_strings = [['1.2', '3.4'], ['5.6', '7.8']]
You can use the following list comprehension:
nested_list_of_floats = [[float(x) for x in inner] for inner in nested_list_of_strings]
This will result in a nested list of floats like [[1.2, 3.4], [5.6, 7.8]]
.
Pheww! Hope this article helped you solve your conversion problems.
Free Cheat Sheets!
If you want to keep learning Python and improving your skills, feel free to check out our Python cheat sheets (100% free):
Be on the Right Side of Change