https://s.w.org/images/core/emoji/14.0.0/72×72/2b50.png
To convert a list of tuples to a Pandas DataFrame, import the pandas
library, call the DataFrame constructor, and pass the list of tuples as the data argument such as in pd.DataFrame(tuples_list, columns=['Number', 'Letter'])
.
Here’s a minimal example:
import pandas as pd tuples_list = [(1, 'A'), (2, 'B'), (3, 'C')] df = pd.DataFrame(tuples_list, columns=['Number', 'Letter'])
The output of the given code will be a Pandas DataFrame with two columns, 'Number'
and 'Letter'
, as follows:
Number Letter
0 1 A
1 2 B
2 3 C
After the Panda image, let’s dive deeper into this conversion technique so you can improve your skills and learn more on Pandas’ assume capabilities!
I’ll also show you how to convert a list of named tuples — and how to convert the DataFrame back to a list of tuples (key-value pairs).

Converting a List of Tuples to DataFrame
First, let’s explore how to convert a list of tuples into a DataFrame using Python’s Pandas library.
Using DataFrame Constructor
The simplest way to convert a list of tuples into a DataFrame is by using the DataFrame()
constructor provided by the Pandas library. This method is straightforward and can be achieved in just a few lines of code.
Here’s an example:
import pandas as pd tuple_list = [('A', 1), ('B', 2), ('C', 3)] df = pd.DataFrame(tuple_list) print(df)
Executing this code will create a DataFrame with the following structure:
0 | 1 |
A | 1 |
B | 2 |
C | 3 |
Handling Data with Column Names
When converting a list of tuples to a DataFrame, it’s often useful to include column names to make the data more readable and understandable. To do this, you can add the columns
parameter when calling the DataFrame()
constructor.
Here’s an example:
import pandas as pd tuple_list = [('A', 1), ('B', 2), ('C', 3)] column_names = ['Letter', 'Number'] df = pd.DataFrame(tuple_list, columns=column_names) print(df)
With the column names specified, the resulting DataFrame will look like this:
Letter | Number |
A | 1 |
B | 2 |
C | 3 |
By using the DataFrame constructor and handling data with column names, you can easily convert a list of tuples into a DataFrame that is more organized and easier to understand. Keep working with these techniques, and soon enough, you’ll be a master of DataFrames!
Examples and Use Cases
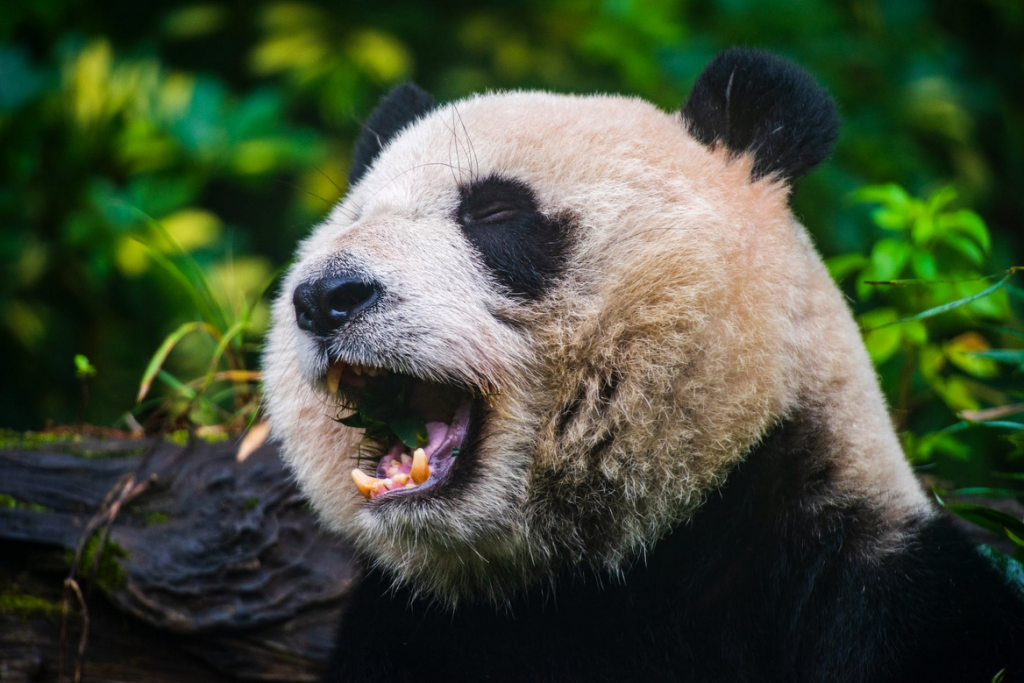
When working with Python, one often encounters data stored in lists of tuples. These data structures are lightweight and easy to use, but sometimes, it’s beneficial to convert them into a more structured format, such as a DataFrame . In this section, we will explore some examples and use cases for converting a list of tuples into a DataFrame in Python, using the pandas library.
Here’s a simple example that demonstrates how to create a DataFrame from a list of tuples:
import pandas as pd data = [('Peter', 18, 7), ('Riff', 15, 6), ('John', 17, 8), ('Michel', 18, 7), ('Sheli', 17, 5)] df = pd.DataFrame(data, columns=['Name', 'Age', 'Score'])
In this example, we have a list of tuples representing student data, with each tuple containing a name, age, and score. By passing this list to the DataFrame constructor along with the column names, we can easily convert it into a DataFrame .
Consider another use case, where we need to filter and manipulate data before converting it into a DataFrame. For instance, let’s imagine we have a list of sales data, with each tuple representing an item, its price, and the number of sales:
data = [('Item A', 35, 20), ('Item B', 45, 15), ('Item C', 50, 30), ('Item D', 25, 10)]
In this case, we can use list comprehensions to filter items with sales greater than 20 and update the price by applying a 10% discount:
filtered_data = [(item, price * 0.9, sales) for item, price, sales in data if sales > 20] df = pd.DataFrame(filtered_data, columns=['Item', 'Discounted Price', 'Sales'])
Now, our DataFrame contains only the filtered items with the discounted prices .
Python List of Named Tuples to DataFrame
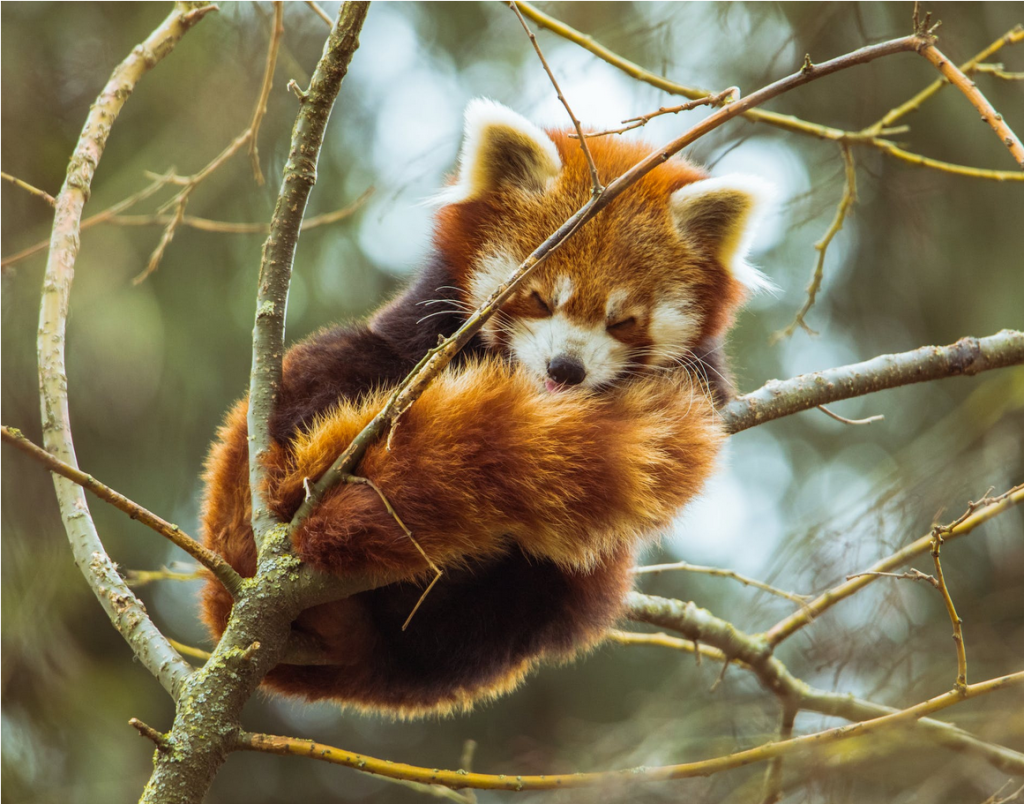
Converting a list of named tuples to a DataFrame in Python can be done efficiently using the pandas
library’s default functions as well.
Info: A named tuple is a subclass of a tuple, which allows you to access elements by name, making it highly readable and practical for data manipulation.
First, create a list of named tuples using Python’s built-in collections
module.
Let’s assume we have a list of students with their names, ages, and test scores:
from collections import namedtuple Student = namedtuple('Student', ['name', 'age', 'score']) students = [ Student('Alice', 23, 89), Student('Bob', 22, 92), Student('Charlie', 24, 85) ]
With the list of named tuples prepared, proceed to import the pandas library and use the pd.DataFrame()
method to convert the list to a DataFrame:
import pandas as pd dataframe = pd.DataFrame(students, columns=Student._fields)
This process creates a DataFrame with columns corresponding to the named tuple fields. The final result appears as follows:
name age score
0 Alice 23 89
1 Bob 22 92
2 Charlie 24 85
In summary, simply define the list with the named tuple structure, and then call the pd.DataFrame()
method to create the DataFrame.
Create a List of Tuples From a DataFrame

When working with data in Python, you may need to convert a DataFrame back into a list of tuples.
To begin, import the library in your Python code using import pandas as pd
.
Now, let’s say you have a DataFrame, and you want to extract its data as a list of tuples. The simplest approach is to use the itertuples()
function, which is a built-in method in Pandas (source).
To use this method, call the itertuples()
function on the DataFrame object, and then pass the output to the list()
function to convert it into a list:
python import pandas as pd # Sample DataFrame data = {'Name': ['John', 'Alice', 'Tim'], 'Age': [28, 22, 27]} df = pd.DataFrame(data) # Convert DataFrame to list of tuples list_of_tuples = list(df.itertuples(index=False, name=None)) print(list_of_tuples)
This code will output:
[('John', 28), ('Alice', 22), ('Tim', 27)]
The itertuples()
method has two optional parameters: index
and name
. Setting index=False
excludes the DataFrame index from the tuples, and setting name=None
returns regular tuples instead of named tuples.
So there you go! You now know how to convert a DataFrame into a list of tuples using the Pandas library in Python . To keep learning and improving your Python skills, feel free to download our cheat sheets and visit the recommended Pandas tutorial:
Recommended: 10 Minutes to Pandas (in 5 Minutes)
Be on the Right Side of Change